[iOS] Custom back button with back gesture
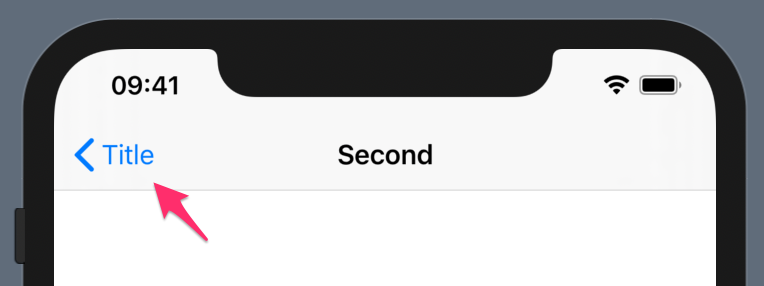
When I want to change the default iOS back button, I need to hide the default
back button. This causes the back gesture is also disabled. Therefore, I need to
keep the back gesture and make the change.
To make these happen, I do steps:
- Disable the default back button
- Set the new back button to left bar item
- Enable the navigation interaction gesture for back gesture
Here is the codes (Swift 5)
class MyViewController: UIGestureRecognizerDelegate {
self.navigationItem.hidesBackButton = true
let image = UIImage(systemName: "chevron.backward")
let backBarItem = UIBarButtonItem(image: image, style: .plain, target: nil, action: nil)
self.navigationItem.leftBarButtonItem = backBarItem
self.navigationController?.interactivePopGestureRecognizer?.delegate = self
func gestureRecognizer(_ gestureRecognizer: UIGestureRecognizer, shouldBeRequiredToFailBy otherGestureRecognizer: UIGestureRecognizer) -> Bool {
return true
}
}
Comments
Post a Comment